-
Key Features and
Create a new project in Visual Studio 2022 - Naming Standards & Comments
- Data Types
- Variables and Constant Variable
- Conditional Statement
- Switch Conditional Statement
- Arithmetic, Comparison, Assignment and Logical Operators
- For, While and Do While Loops
- Importance of Break and Continue Keywords
- Strings and Important String Methods
- Single Dimensional Array
- Multi Dimensional Array
- Methods and Method Overloading
- Math Class and Functions
- Casting and Type Conversions
- Class and Objects
- Constructor, Parameter less and Parameterized Constructor
- Single, Multi level and Hierarchical Inheritance
- Compile Time Polymorphism, Method and Operator Overloading
- Run-time Polymorphism and Importance of base keyword
- Abstract Class and Method
- Interface and Multiple Inheritance through Interfaces
Agenda
- Inheritance in C#
- Single Inheritance
- Multi level Inheritance
- Hierarchical Inheritance
- Multiple Inheritance
Inheritance in C#
- Inheritance is a feature of object-oriented programming (OOP) languages that allows user to define a base class that provides specific functionality (data and behavior) and to define derived classes that either inherit or override that functionality.
- Using Inheritance user can create Classes that are built in upon existing classes.
- When user Inherit from an existing class, then they can reuse Methods and fields from Parent class and can add new methods and fields as well.
- The class where the class members are getting Inherited is called as Super class/Parent class/Base class.
- The class to which the class members are getting Inherited is called as Sub class/Child class/Derived class.
- To inherit from a class, user can use the : symbol
Types of Inheritance in C#:
1) Single Inheritance:
A single derived class inherits from a single base class
public class ClassB : ClassA {
}
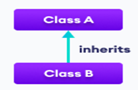
2) Multi level Inheritance:
A derived class inherits from a base and then the same derived class acts as a base class for another class.
public class ClassB : ClassA {
}
public class ClassC : ClassB {
}
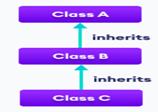
Types of Inheritance in C#
3) Hierarchical Inheritance:
One class serves as a superclass (base class) for more than one subclass.
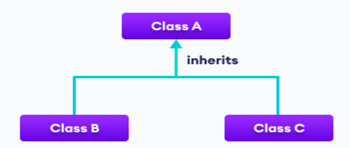
Multiple Inheritance ( Through Interfaces Only)
One class can have more than one superclass and inherit features from all parent classes. As C# does not support multiple inheritance with classes, user can achieve multiple inheritance only through Interfaces in C#.
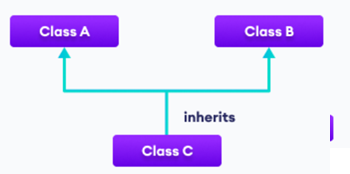
C# Single and Multi-level Inheritance
public class ClassA
{ public int a = 20;
public void add(int x, int y)
{
Console.WriteLine(x + y);
} }
public class ClassB : ClassA
{ public int b = 30;
public void substraction(int x, int y)
{
Console.WriteLine(x - y);
} }
public class ClassC : ClassB
{ public int c = 30;
public void multiplication(int x, int y)
{
Console.WriteLine(x * y);
} }
static void Main() {
ClassA objA = new ClassA();
Console.WriteLine(objA.a); objA.add(10, 20);ClassB objB = new ClassB();
Console.WriteLine(objB.a); objB.add(30, 20);
Console.WriteLine(objB.b); objB.substraction(30, 20);
ClassC objC = new ClassC();
Console.WriteLine(objC.a); objC.add(30, 20);
Console.WriteLine(objC.b); objC.substraction(30, 20);
Console.WriteLine(objC.c); objC.multiplication(30, 20);
}
Object Oriented Programming Structure
Inheritance
Polymorphism
Encaptulation
Abstractionpublic class ClassA
{
public int a = 20;
public void add(int x, int y)
{
Console.WriteLine(x + y);
}
}
public class ClassD : ClassA
{
public int d = 50;
public void division(int x, int y)
{
Console.WriteLine(x/y);
}
}
public class ClassB : ClassA
{
public int b = 30;
public void substraction(int x, int y)
{
Console.WriteLine(x - y);
}
}
public class ClassC : ClassB
{
public int c = 30;
public void multiplication(int x, int y)
{
Console.WriteLine(x * y);
}
}
ClassA objA = new ClassA();
Console.WriteLine(objA.a);
objA.add(10, 20);
ClassB objB = new ClassB();
Console.WriteLine(objB.a);
objB.add(30, 20);
Console.WriteLine(objB.b);
objB.substraction(30, 20);// Console.WriteLine(objB.a); error while accessing Class a variable without Inheritance
ClassC objC = new ClassC();
Console.WriteLine(objC.a);
objC.add(30, 20);
Console.WriteLine(objC.b);
objC.substraction(30, 20);
Console.WriteLine(objC.c);
objC.multiplication(30, 20);ClassD objD = new ClassD();
Console.WriteLine(objD.a);
objD.add(30, 20);
Console.WriteLine(objD.d);
objD.division(30, 10);